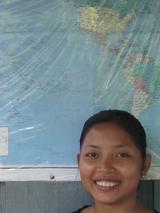 |
Web frameworks are development environments that make it faster and easier to build quality web apps. Many frameworks exist, including ASP.NET and Java Struts. Different frameworks have radically different approaches, resulting in a variety of strengths and weaknesses. Recently there has been particular interest around frameworks in dynamic languages, where Ruby on Rails is a pioneer.
Before Frameworks
Before frameworks, many web apps were coded in languages like PHP, JSP and ASP. These languages make it easy to embed code in a web page, but don't offer much further support. Regardless of the language used, most web apps use an SQL database to store data on the server. This arrangement certainly works, and has supported major websites for several years, but there are some problems:
- Code and presentation is tied together; a designer who doesn't program cannot easily update the look and feel.
- Database access uses embedded SQL, and requires quite a lot of code.
- A lot of code is required for helpfully validating form data.
- Pages are independent; it is difficult to do site-wide tasks, such as checking for a login session.
- Common components (e.g. a popup calendar) require some work to integrate.
- Considerable knowledge and care is required to avoid security vulnerabilities.
MVC Architecture
Most frameworks are based on a Model-View-Controller (MVC) architecture. Roughly speaking, this means code is separated depending on whether it is to do with data storage (model), presentation (view) or everything else (controller). Frameworks vary in the precise definition of each component, and the degree to which this architecture is followed. In general, this is a very helpful way of dividing an application into components.
To take a contrasting example, most PHP applications have no separation between the model, view and controller - each page contains some code relating to all three.
Other Features
As well as helping to structure your application in a useful way, most frameworks try to offer convenient ways to achieve common developer requirements. Some of the more common ones are:
- Authentication and authorisation, user registration, forgotten passwords, etc.
- Reusable components - popup calendars, rich text editors, etc.
- Libraries for form generation and validation; automatic construction from a database model.
- JavaScript libraries and server-side code to work with JSON.
- Tools to assist with deployment and management of a live application.
- Internationalisation and localisation.
Model - Object-Relational Mapper
Most frameworks encourage the use of an object-relational mapper (ORM) for the model. An ORM provides an object-orientated interface to an SQL database, with classes representing tables, and class instances representing rows. This has a number of benefits:
- The data classes provide a convenient place to put code relating to data storage.
- Common database uses can be achieved in much less code than embedded SQL, and this helps avoid a conceptual shift between program code and SQL.
- The ORM can offer advanced functionality, such as polymorphism and sharding.
- The data classes act as a master definition for data structure in the application.
- Most ORMs provide portability across a variety of SQL databases (Oracle, MySQL, etc.).
ORMs do not suit all applications. If there is a lot of complex data querying, the ORM may do no more than add another layer of complexity, and using plain SQL would be appropriate. An MVC architecture does not enforce use of an ORM, but they do work well together.
View - Template Languages
Most frameworks use a templating language for the view. This is generally HTML with some extra annotations. For example, in Genshi templates, py:for can be used to do a for loop. These annotations are processed on the server, so the client only sees regular HTML. Templating languages generally have the following features:
- Variable substitution - e.g. $username is replaced by the username variable.
- Loops (for) and conditional blocks (if).
- Embedding expressions or code, e.g. ${username.lower()}
- Reusable blocks, somewhat like functions.
Some template languages work only with XML-based files, e.g. XSLT. Others work with any text file, e.g. Cheetah. Some deliberately restrict the ability to include code, e.g. Django, while most allow arbitrary code. Most template languages are bound to a programming language, but some are general, e.g. Clearsilver.
Controller
For a web framework, the main function of a controller is to deliver web requests to application code. Many languages have a common interface to web servers (e.g. the Java Servlet interface). Frameworks can utilise this to support all web servers that can interface with their language. In addition, most frameworks have a built-in web server, which is convenient for development as it can be run without configuration.
There are several approaches to translating web requests to code calls. One approach is to treat functions somewhat like pages - a request to /myfunc results in myfunc() being called. Another approach is to have a definition table that provides rules to map URLs to functions. These approaches are useful in different applications; many frameworks offer the user a choice.
|